Last fall I worked on a demo project for IoT device tracking on AWS so I had some reading.
Overview
From a strategic level, as servers move away to the cloud, AWS envisions that the footprint left on premise will mainly be IoT devices. The role of the cloud therefore becomes a central point of management for IoT devices. As a result, most of the AWS services for IoT are managed services. The best resources are two white papers: IoT Lens – AWS Well-Architected Framework and Securing Internet of Things (IoT) with AWS. The most important services are:
- IoT Analytics: Makes it easy to run sophisticated analytics on volumes on IoT data. It connects to the underlying IoT data store and allow you to build your own analytical queries and Jupiter notebooks.
- IoT Events
- IoT Core: Core features for IoT.
- IoT SiteWise
- IoT Device Management
- IoT Greengrass
- FreeRTOS: IoT devices usually need to be small and power efficient. The software and OS running on the device is important. FreeRTOS is a real-time operating system for micro-controllers supported by AWS. FreeRTOS provides kernel, OS and libraries to securely connect your edge device to the cloud in no time.
In this post I will explore IoT architecture at high level. In real life, you program your device with AWS IoT Device SDK and AWS IoT API in different languages. In this post, I use a script to simulate GPS data, and push it to AWS IoT using SDK. Then I render the location using sample AWS code for Amazon Location.
IoT Architecture
When creating IoT services we consider registration and telemetry capturing flow. The Well architected white paper proposes registration flow as such:
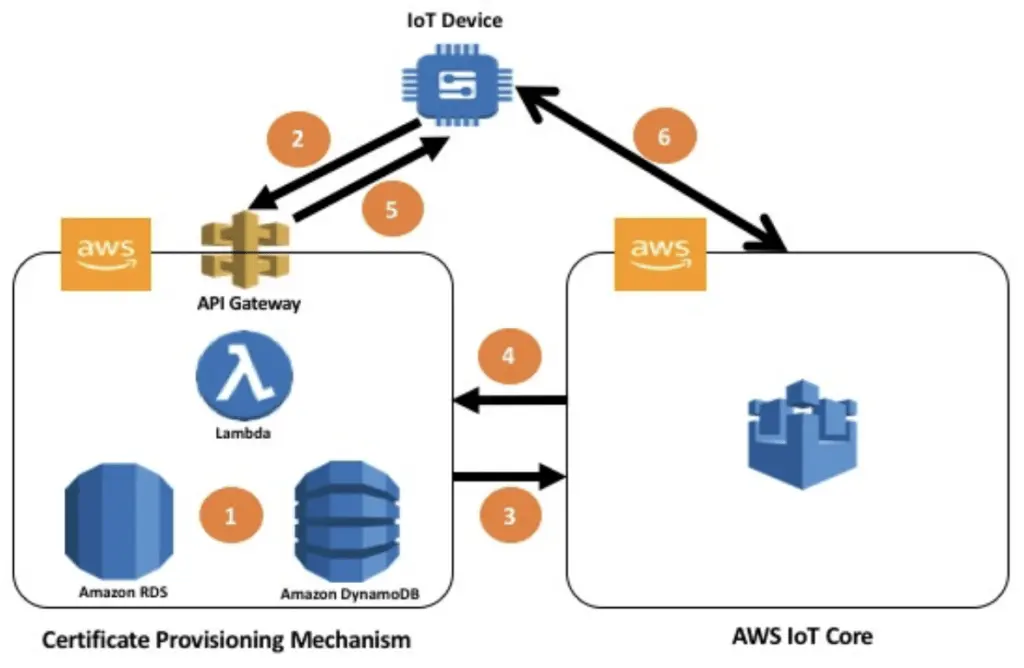
Device Registry to keep track of devices (aka Things). You can find where your devices are, and filter by a common feature (e.g. ModelX device only). Registration flow usually involves a testing of communication between device and server. The authentication must be 2-way where server needs to validate device identity, and device needs to validate server identity. You can use a unique X.509 certificate per device to adhere to security best practices on AWS. This way, if one device gets hacked, the entire fleet of devices is not affected by one certificate being compromised. An alternative authentication method is Cognito. With Cognito you can sign your users into a mobile application, so you use IAM policies to authenticate them into viewing different dashboards or viewing the data that pertains to them specifically. IoT Core policies can help manage authorization.
The white paper also proposes a few options for capturing telemetry:
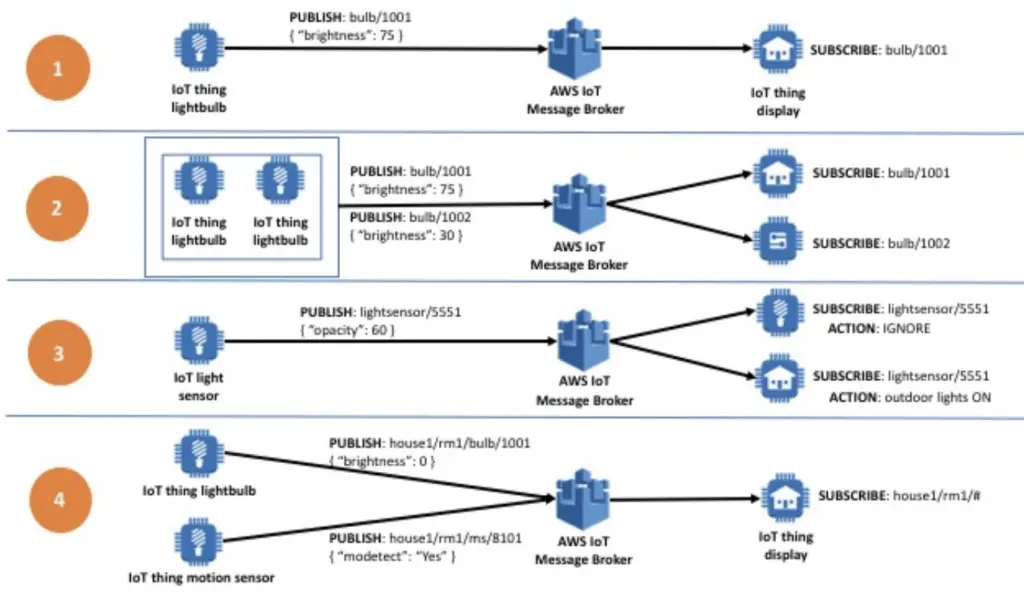
These options presents a common pub-sub pattern, where the device streams message by topic to the Message Broker in IoT core. The IoT core also involves policy and rules. A rule may involve a subscriber to consume the messages. A more comprehensive architecture from AWS IoT device connectivity workshop looks like this:
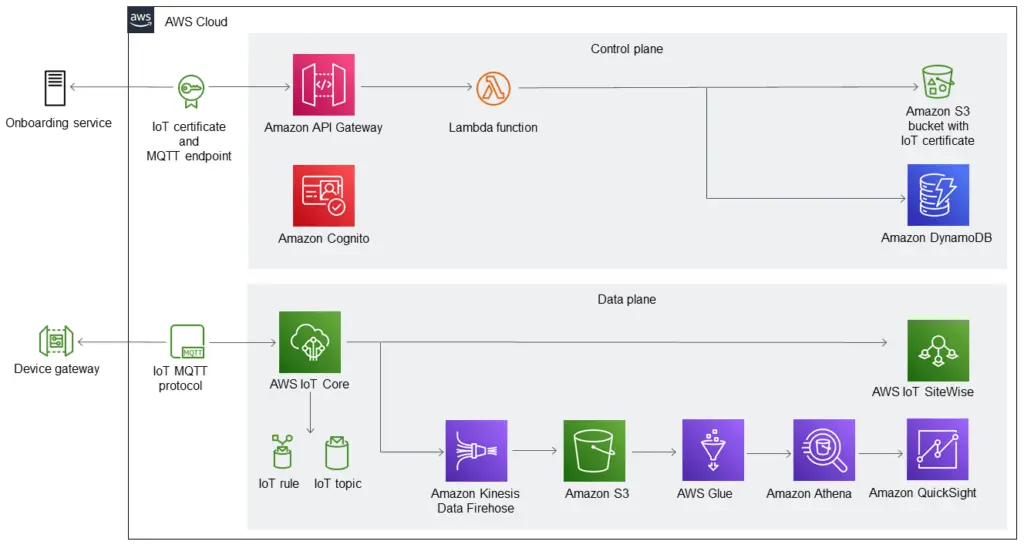
The message path from device to IoT core remains the same. When building an IoT solution, we first address the messaging path.
IoT Protocols
In IoT core, Device Gateway is the entry point for IoT devices connecting to AWS.It supports MQTT, WebSockets and HTTP 1.1 protocols, on top of TLS. Registration flow uses HTTP/REST protocol for provisioning, and with MQTT protocol for a message test. For pushing telemetry, we can use both but prefer MQTT (topic based) because of its advantages in IoT messaging. Here is a good article on the differences.
AWS has a white paper on designing MQTT topics, with a few communication patterns and best practices. The SDK documentation also explained the communication protocols, including:
- HTTPS: publish only
- MQTT: publish and subscribe
- MQTT over WebSocket: publish and subscribe. Device Gateway will maintain long lived, bi-directional connections, enabling devices to send and receive messages at any time with low latency.
Pay attention to the authentication mechanism. As to what protocol is used in a communication, they can be dynamically negotiated using the ALPN protocol. ALPN (Application-Layer Protocol Negotiation) is a TLS protocol extension that allows the application layer to negotiate which protocol should be performed over a secure connection in a manner that avoids additional round trips and which is independent of the application-layer protocols.
GPS data simulator
I don’t have a GPS chip. To get sample GPS data, I used geojson.io website, use a pen to paint the points and collect the result in JSON format. The data looks like this:
{
"type": "FeatureCollection",
"features": [
{
"type": "Feature",
"properties": {},
"geometry": {
"coordinates": [
[
-119.4966304331144,
49.88901098598916
],
[
-119.4966304331144,
49.889903059931726
],
[
-119.49658503509582,
49.89066350338888
],
[
-119.49658503509582,
49.891365440561145
],
[
-119.4966304331144,
49.89219897769374
],
[
-119.4966304331144,
49.88735841200943
],
[
-119.49660773410511,
49.8880603972496
],
[
-119.4966304331144,
49.88885011844141
]
],
"type": "LineString"
}
}
]
}
Save this file as map.geojson to later feed it to device simulation script.
Rendering location data
In AWS location samples project, the sample project maplibre-js-react-iot-asset-tracking is a good demo of IT. The readme document contains a walk through, using AWS amplify services. The steps includes creating certificates, configuring lambda function to add location data to tracker. The project directory also includes the device simulation script, as index.js. I slightly modified the content to this:
const awsIot = require("aws-iot-device-sdk");
// Replace with your AWS IoT endpoint
const THING_ENDPOINT = "safdsa-ats.iot.us-east-1.amazonaws.com"; // get from console
const CLIENT_ID = "trackThing01";
const IOT_TOPIC = "iot/trackedAssets";
const DEVICE_ID = "thing123";
const GEOJSON_FILEPATH="geojson/map.geojson";
const fs = require('fs')
const file_raw = fs.readFileSync(GEOJSON_FILEPATH).toString();
const positions_raw = JSON.parse(file_raw).features[0].geometry.coordinates
const POINTS_ON_MAP=[]
for (var i=0;i<positions_raw.length;i++){
POINTS_ON_MAP.push({lat:positions_raw[i][1],long:positions_raw[i][0]})
}
const device = awsIot.device({
host: THING_ENDPOINT,
keyPath: `${__dirname}/certs/private.pem.key`,
certPath: `${__dirname}/certs/certificate.pem.crt`,
caPath: `${__dirname}/certs/root-CA.pem`,
clientId: CLIENT_ID,
keepalive: 60000,
});
console.log("Connecting to %s with client ID %s", THING_ENDPOINT, CLIENT_ID);
device.on("connect", async function () {
console.log("Connected to device %s", CLIENT_ID);
for (const point of POINTS_ON_MAP) {
const message = {
payload: {
deviceId: DEVICE_ID,
timestamp: new Date().getTime(),
location: point,
},
};
console.log(
"Publishing message to topic %s: %s",
IOT_TOPIC,
JSON.stringify(message)
);
device.publish(IOT_TOPIC, JSON.stringify(message), { qos: 1 });
// Set timeout to sleep
await new Promise((resolve) => setTimeout(resolve, 10000));
}
device.end();
});
We can obtain certificate ID from AWS console or by CLI command:
aws iot list-certificates --output text --query 'reverse(sort_by(certificates,&creationDate))[:1].[certificateId]' | cat
When running the script, it pushes data to IoT core service. The AWS Amplify project creates Lambda function that is subscribed to the topic and trigger actions. The data are used to render points on a map, which is available on the front end.
Summary
This is an overly simplified use case but it covers the basics. IoT solution will use a lot managed service and familiar technologies (e.g. TLS, certificate). Creating an IoT solution is mostly about address the onboarding services and make use of the MQTT based workflow. AWS managed services makes these easier.

More IoT workshops are available on AWS workshops.